Documentation
Our guides to integrate with Weavr.
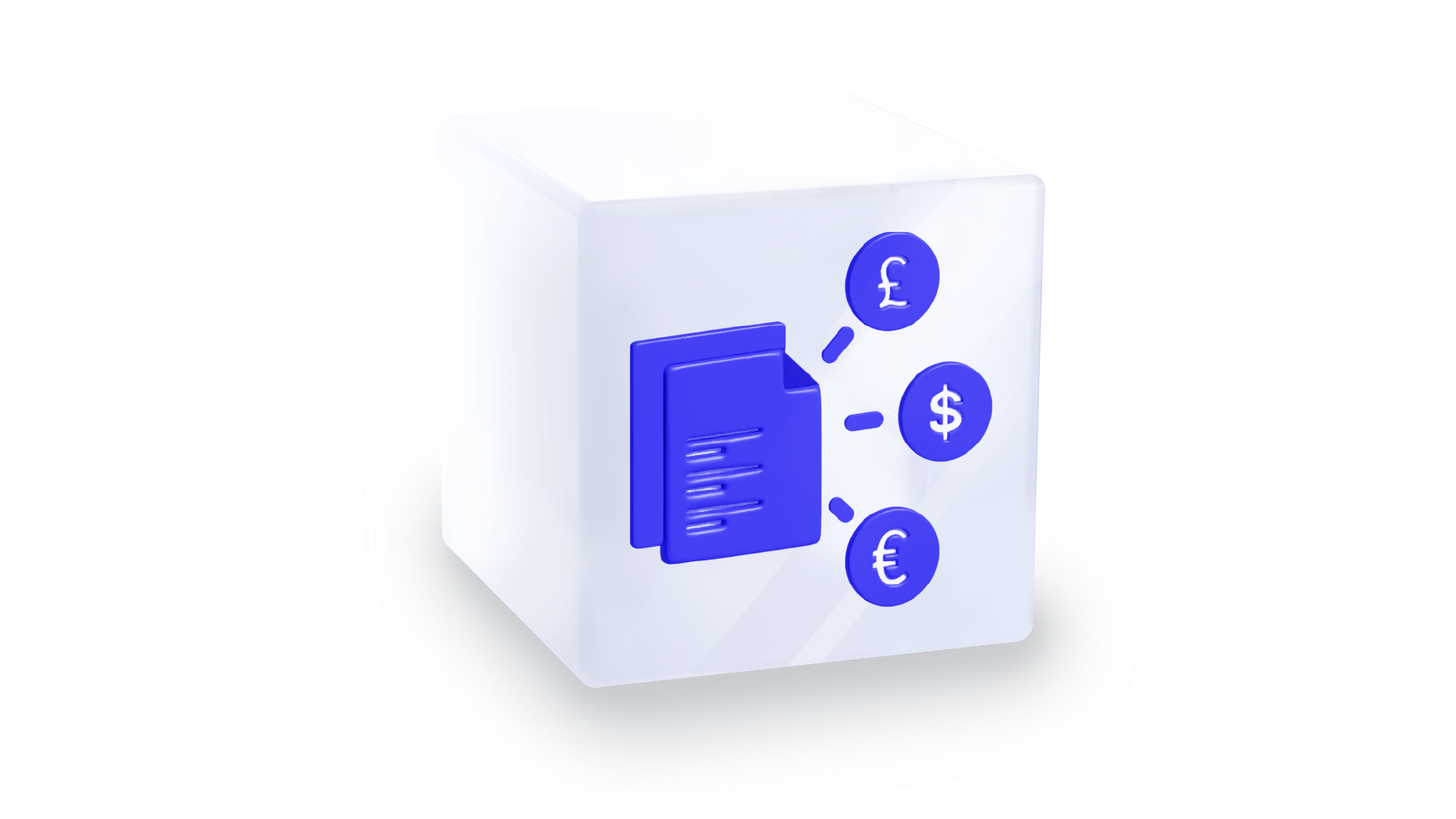
Payment Run
- Bulk payments for AP apps
- Specially tailored API
- Simplified, faster setup programme
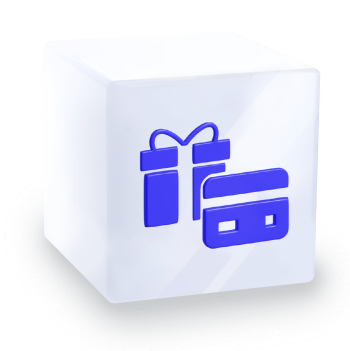
Flexible Benefits
- Spendable benefits for employees on virtual or physical cards
- Benefits-oriented controls for employers
- Comprehensive benefit management solutions
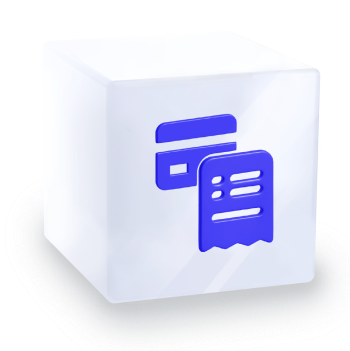
Smarter Expenses
- Employees spend within defined rules on virtual or physical cards
- Expenses-oriented controls for employers
- Replaces traditional expense claim / reimbursement paradigm
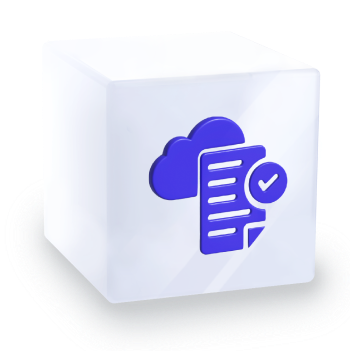
Smarter IT Bill Payments
- Virtual cards for IT and SaaS administrators to control software and cloud spend
- Subscription-oriented controls for IT and finance management
- Centralisation of billing helps stamp out shadow IT
COMING SOON
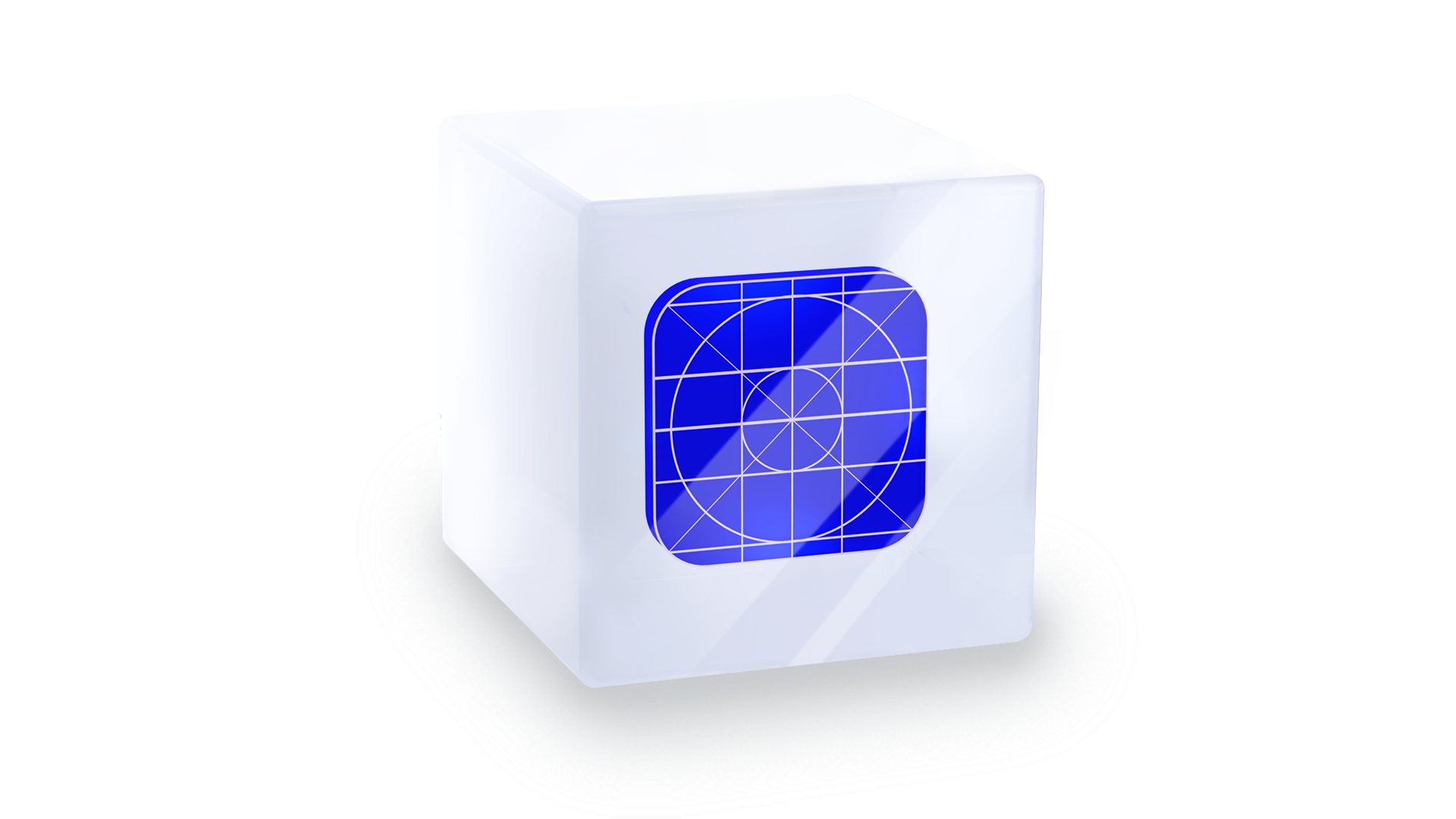